Hive Developer Portal
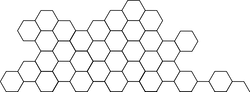
Paginated API Methods
Querying multiple pages of results from the API
Intro
Typically, the initial request from API calls will be sufficient for showing what’s happening in the blockchain right now. But often, it is desirable to reach further back in time.
For web-based applications, it is ideal to show the initial page of results, followed by older results as needed.
The purpose of pagination is to limit the time it takes to return the most relevant results, making each page of results easier to handle.
The typical maximum result for one page of results is 1,000 objects (with some exceptions).
Note: For these examples, we are going to use a command-line JSON processor called jq
to interpret JSON results.
apt-get install jq
Instead of jq
, you could also pipe to python -mjson.tool
, as an alternative.
Limit
Here, we are setting a limit of 10:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", ""],
"limit": 10,
"order": "by_comment_voter"
},
"id": 1
}' https://api.hive.blog | jq
Start (primarily database_api.list_*
)
Extending the previous example, if we wanted to get the next page of results, we can provide values in start
by using the last result of our previous request:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["steemit", "firstpost", "red"],
"limit": 10,
"order": "by_comment_voter"
},
"id": 1
}' https://api.hive.blog | jq
Using this pattern, it is possible to get the entire list of votes, given enough time.
Order (primarily database_api.list_*
)
Extending the previous example, the order
of results declares the structure of start
. So if we request order
of by_comment_voter
, then start
must be author
, permlink
, voter
.
But if we change the order to by_voter_comment
, then start
must be voter
, author
, permlink
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["red", "steemit", "firstpost"],
"limit": 10,
"order": "by_comment_voter"
},
"id": 1
}' https://api.hive.blog | jq
Using by_comment_voter
sorts by the author/permlink
, then the voter
, so the start param consists of author
, permlink
, voter
.
The author
value is the author of the comment, permlink
value is the permlink
of the comment, and voter
value is the voter to start listing from. For example, ["steemit", "firstpost", ""]
will list, in order, the vote objects on the steemit/firstpost
post, starting from the beginning since we did not supply a voter
in the start param.
On the other hand, by_voter_comment
returns the votes a particular voter cast, starting from the provided permlink
, thus the start param requires voter
, author
, permlink
. For example, ["red", "", ""]
, will list, in order, the vote objects cast by voter red
and will start from the beginning because no author/permlink
was provided. The results will be every single vote object associated with either the comment or the voter.
If you want only the votes cast by, for example, steemit
since the last vote on steemit/firstpost
then use, ["steemit", "steemit", "firstpost"]
with order by_voter_comment
.
Sections
The following methods have various forms of pagination:
account_history_api
database_api
list_accounts
list_change_recovery_account_requests
list_comments
list_decline_voting_rights_requests
list_escrows
list_limit_orders
list_owner_histories
list_savings_withdrawals
list_hbd_conversion_requests
list_vesting_delegation_expirations
list_vesting_delegations
list_votes
list_withdraw_vesting_routes
list_witness_votes
list_witnesses
list_proposal_votes
list_proposals
list_smt_contributions
list_smt_token_emissions
list_smt_tokens
follow_api
reputation_api
tags_api
condenser_api
account_history_api.get_account_history
Although the name of the param is start
, it’s better to think of it as from
. We are telling the API that we would like to read from the nth object minus the limit
. Like all limit
params in the API, get_account_history
has a limit of 1,000 objects.
Note: The start
param may not be less than limit
. The start
param may also be negative (-1
).
The following asks for the first 10 objects in account history ("start": 10
):
curl -s --data '{
"jsonrpc": "2.0",
"method": "account_history_api.get_account_history",
"params": {
"account": "steemit",
"start": 10,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To get the next page of objects ("start": 20
):
curl -s --data '{
"jsonrpc": "2.0",
"method": "account_history_api.get_account_history",
"params": {
"account": "steemit",
"start": 20,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To get the latest 10 objects ("start": -1
):
curl -s --data '{
"jsonrpc": "2.0",
"method": "account_history_api.get_account_history",
"params": {
"account": "steemit",
"start": -1,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_accounts
This method allows three different values for the order
param:
by_name
-start
requiresaccount
by_proxy
-start
requires 2 values:account
,account
by_next_vesting_withdrawal
-start
requires 2 values:timestamp
,account
To list the first 10 accounts by name, starting from the very first account:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": "",
"limit": 10,
"order": "by_name"
},
"id":1
}' https://api.hive.blog | jq
To list the next page of accounts by name (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": "alice",
"limit": 10,
"order": "by_name"
},
"id":1
}' https://api.hive.blog | jq
When using order
of by_proxy
, the start
param must be an array with the first element of the account being proxied to and the second being the first account to page from. Thus, to get the first page of accounts that proxy to alice
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": ["alice", ""],
"limit": 10,
"order": "by_proxy"
},
"id":1
}' https://api.hive.blog | jq
Then, to get the second page, assuming bob
is the last account in the first result:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": ["alice", "bob"],
"limit": 10,
"order": "by_proxy"
},
"id":1
}' https://api.hive.blog | jq
Note: Using order
of by_proxy
only orders the objects by proxy, it does not filter them (nor should it).
To list the first 10 accounts by next vesting withdrawal (powerdown):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": ["2019-09-11T00:00:00", ""],
"limit": 10,
"order": "by_next_vesting_withdrawal"
},
"id":1
}' https://api.hive.blog | jq
To list the next page of accounts by next vesting withdrawal:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_accounts",
"params": {
"start": ["2019-09-11T00:25:33"],
"limit": 10,
"order": "by_next_vesting_withdrawal"
},
"id":1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_change_recovery_account_requests
This method allows two different values for the order
param:
by_account
-start
requiresaccount
by_effective_date
-start
requires 2 values:timestamp
,account
To list the first 10 accounts by name with an relevant recovery account request:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_change_recovery_account_requests",
"params": {
"start": "",
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of accounts by name (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_change_recovery_account_requests",
"params": {
"start": "alice",
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 accounts by effective date then by name with an relevant recovery account request:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_change_recovery_account_requests",
"params": {
"start": ["2019-09-11T00:25:33",""],
"limit": 10,
"order": "by_effective_date"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_comments
by_cashout_time
-start
requires 3 values:timestamp
,author
,permlink
by_permlink
-start
requires 2 values:author
,permlink
by_root
-start
requires 4 values:root_author
,root_permlink
,child_author
,child_permlink
by_parent
-start
requires 4 values:child_author
,child_permlink
,root_author
,root_permlink
by_last_update
-start
requires 4 values:parent_author
,update_time
,start_author
,permlink
by_author_last_update
-start
requires 4 values:parent_author
,update_time
,start_author
,permlink
To list the first 10 posts/comments by cashout time:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["1970-01-01T00:00:00", "", ""],
"limit": 10,
"order": "by_cashout_time"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by cashout time (assuming 2019-09-11T03:13:03
, alice
, alice-permlink
is the last timestamp
, author
, permlink
entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["2019-09-11T03:13:03", "alice", "alice-permlink"],
"limit": 10,
"order": "by_cashout_time"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 posts/comments by permlink:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["", ""],
"limit": 10,
"order": "by_permlink"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by permlink (assuming alice
, alice-permlink
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["alice", "alice-permlink"],
"limit": 10,
"order": "by_permlink"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 posts/comments by root post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "announcing-the-launch-of-hive-blockchain", "", ""],
"limit": 10,
"order": "by_root"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by root post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "announcing-the-launch-of-hive-blockchain", "traducciones", "q7d314"],
"limit": 10,
"order": "by_root"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 posts/comments by parent post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "announcing-the-launch-of-hive-blockchain", "", ""],
"limit": 10,
"order": "by_parent"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by parent post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "announcing-the-launch-of-hive-blockchain", "tsnaks", "re-hiveio-q7d32c"],
"limit": 10,
"order": "by_parent"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 posts/comments by last update:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "2020-03-20T00:00:00", "", ""],
"limit": 10,
"order": "by_last_update"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by parent post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "2020-03-20T00:00:00", "titusfrost", "q7g5ef"],
"limit": 10,
"order": "by_last_update"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 posts/comments by author last update:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "2021-03-20T00:00:00", "", ""],
"limit": 10,
"order": "by_author_last_update"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of posts/comments by parent post:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_comments",
"params": {
"start": ["hiveio", "2021-03-20T00:00:00", "hiveio", "final-hive-hf24-date-set-october-6-2020"],
"limit": 10,
"order": "by_author_last_update"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_decline_voting_rights_requests
by_account
-start
requires:account
by_effective_date
-start
requires 2 values:timestamp
,account
To list the first 10 accounts:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_decline_voting_rights_requests",
"params": {
"start": "",
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of accounts (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_decline_voting_rights_requests",
"params": {
"start": "alice",
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 accounts by effective date:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_decline_voting_rights_requests",
"params": {
"start": ["1970-01-01T00:00:00", ""],
"limit": 10,
"order": "by_effective_date"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of accounts (assuming 2019-09-12T00:00:00
, alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_decline_voting_rights_requests",
"params": {
"start": ["2019-09-12T00:00:00", "alice"],
"limit": 10,
"order": "by_effective_date"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_escrows
by_from_id
-start
requires 2 values:account
,escrow_id
by_ratification_deadline
-start
requires 3 values:is_approved
,timestamp
,escrow_id
To list the first 10 escrows ordered by from
, escrow_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_escrows",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of accounts (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_escrows",
"params": {
"start": ["alice", 99],
"limit": 10,
"order": "by_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 escrows ordered by ratification_deadline
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_escrows",
"params": {
"start": [true, "1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_ratification_deadline"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of escrows (assuming true
, 2019-09-12T00:00:00
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_escrows",
"params": {
"start": [true, "2019-09-12T00:00:00", 99],
"limit": 10,
"order": "by_ratification_deadline"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_limit_orders
by_price
-start
requires 2 values:price
,order_type
by_account
-start
requires 2 values:account
,order_id
To list the first 10 limit ordered by price
, order_type
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_limit_orders",
"params": {
"start": [],
"limit": 10,
"order": "by_price"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of limit orders (assuming base
of 85.405 HIVE
, quote
of 17.192 HBD
is the last price entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_limit_orders",
"params": {
"start": [{
"base": {"amount": "85405", "precision": 3, "nai": "@@000000021"},
"quote": {"amount": "17192", "precision": 3, "nai": "@@000000013"}
}, 0],
"limit": 10,
"order": "by_price"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 limit ordered by account
, order_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_limit_orders",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of limit orders (assuming alice
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_limit_orders",
"params": {
"start": ["alice", 1567828370],
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_owner_histories
Has no order
param. To list the first 10 owner histories ordered by last_valid_time
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_owner_histories",
"params": {
"start": ["", "1970-01-01T00:00:00"],
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of owner histories (assuming alice
, 2019-09-12T00:00:00
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_owner_histories",
"params": {
"start": ["alice", "2019-09-12T00:00:00"],
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_savings_withdrawals
by_from_id
-start
requires 2 values:account
,request_id
by_complete_from_id
-start
requires 3 values:timestamp
,account
,request_id
by_to_complete
-start
requires 3 values:account
,timestamp
,order_id
To list the first 10 withdrawals ordered by from
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of withdrawals (assuming alice
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["alice", 1567828370],
"limit": 10,
"order": "by_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 withdrawals ordered by completed
, from
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["1970-01-01T00:00:00", "", 0],
"limit": 10,
"order": "by_complete_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of withdrawals (assuming 2019-09-12T00:00:00
, alice
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["2019-09-12T00:00:00", "alice", 1567828370],
"limit": 10,
"order": "by_complete_from_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 withdrawals ordered by to
, completed
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["", "1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_to_complete"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of withdrawals (assuming alice
, 2019-09-12T00:00:00
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_savings_withdrawals",
"params": {
"start": ["alice", "2019-09-12T00:00:00", 1567828370],
"limit": 10,
"order": "by_to_complete"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_hbd_conversion_requests
by_account
-start
requires 2 values:account
,request_id
by_conversion_date
-start
requires 2 values:timestamp
,request_id
To list the first 10 conversions ordered by from
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_hbd_conversion_requests",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of conversions (assuming alice
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_hbd_conversion_requests",
"params": {
"start": ["alice", 1567828370],
"limit": 10,
"order": "by_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 conversions ordered by from
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_hbd_conversion_requests",
"params": {
"start": ["1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_conversion_date"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of conversions (assuming 2019-09-12T00:00:00
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_hbd_conversion_requests",
"params": {
"start": ["2019-09-12T00:00:00", 1567828370],
"limit": 10,
"order": "by_conversion_date"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_vesting_delegation_expirations
by_expiration
-start
requires 2 values:timestamp
,expiration_id
by_account_expiration
-start
requires 3 values:account
,timestamp
,expiration_id
To list the first 10 delegation expirations ordered by expiration
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegation_expirations",
"params": {
"start": ["1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_expiration"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of delegation expirations (assuming 2019-09-12T00:00:00
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegation_expirations",
"params": {
"start": ["2019-09-12T00:00:00", 1567828370],
"limit": 10,
"order": "by_expiration"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 delegation expirations ordered by account
, expiration
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegation_expirations",
"params": {
"start": ["", "1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_account_expiration"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of delegation expirations (assuming alice
, 2019-09-12T00:00:00
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegation_expirations",
"params": {
"start": ["alice", "2019-09-12T00:00:00", 1567828370],
"limit": 10,
"order": "by_account_expiration"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_vesting_delegations
by_delegation
-start
requires 2 values:delegator
,delegatee
To list the first 10 delegations ordered by delegations
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegations",
"params": {
"start": ["", ""],
"limit": 10,
"order": "by_delegation"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of delegations (assuming alice
, bob
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_vesting_delegations",
"params": {
"start": ["alice", "bob"],
"limit": 10,
"order": "by_delegation"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_votes
by_comment_voter
-start
requires 3 values:author
,permlink
,voter
by_comment_voter_symbol
-start
requires 3 or 4 values:author
,permlink
,voter
,symbol
by_voter_comment
-start
requires 3 values:voter
,author
,permlink
by_voter_comment_symbol
-start
requires 3 or 4 values:voter
,author
,permlink
,symbol
by_comment_symbol_voter
-start
requires 2 or 4 values:author
,permlink
,symbol
,voter
by_voter_symbol_comment
-start
requires 2 or 4 values:voter
,symbol
,author
,permlink
To list the first 10 votes ordered by comment
, voter
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", ""],
"limit": 10,
"order": "by_comment_voter"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["steemit", "firstpost", "red"],
"limit": 10,
"order": "by_comment_voter"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by comment
, voter
, symbol
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", "", ""],
"limit": 10,
"order": "by_comment_voter_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["steemit", "firstpost", "red", "HIVE"],
"limit": 10,
"order": "by_comment_voter_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by voter
, comment
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", ""],
"limit": 10,
"order": "by_voter_comment"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["nxt2", "dan", "is-the-dao-going-to-be-doa"],
"limit": 10,
"order": "by_voter_comment"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by voter
, comment
, symbol
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", "", ""],
"limit": 10,
"order": "by_voter_comment_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["nxt2", "dan", "is-the-dao-going-to-be-doa", "HIVE"],
"limit": 10,
"order": "by_voter_comment_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by comment
, symbol
, voter
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", "", ""],
"limit": 10,
"order": "by_comment_symbol_voter"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["dan", "is-the-dao-going-to-be-doa", "HIVE", "nxt2"],
"limit": 10,
"order": "by_comment_symbol_voter"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by voter
, symbol
, comment
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["", "", "", ""],
"limit": 10,
"order": "by_voter_symbol_comment"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_votes",
"params": {
"start": ["nxt2", "HIVE", "dan", "is-the-dao-going-to-be-doa"],
"limit": 10,
"order": "by_voter_symbol_comment"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_withdraw_vesting_routes
by_withdraw_route
-start
requires 2 values:from_account
,to_account
by_destination
-start
requires 2 values:to_account
,route_id
To list the first 10 routes ordered by from_account
, to_account
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_withdraw_vesting_routes",
"params": {
"start": ["", ""],
"limit": 10,
"order": "by_withdraw_route"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of routes (assuming alice
, bob
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_withdraw_vesting_routes",
"params": {
"start": ["alice", "bob"],
"limit": 10,
"order": "by_withdraw_route"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 routes ordered by to_account
, route_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_withdraw_vesting_routes",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_destination"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of routes (assuming bob
, 1567828370
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_withdraw_vesting_routes",
"params": {
"start": ["bob", 1567828370],
"limit": 10,
"order": "by_destination"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_witness_votes
by_account_witness
-start
requires 2 values:account
,witness
by_witness_account
-start
requires 2 values:witness
,account
To list the first 10 votes ordered by account
, witness
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witness_votes",
"params": {
"start": ["", ""],
"limit": 10,
"order": "by_account_witness"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes (assuming alice
, bob
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witness_votes",
"params": {
"start": ["alice", "bob"],
"limit": 10,
"order": "by_account_witness"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered by account
, witness
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witness_votes",
"params": {
"start": ["", ""],
"limit": 10,
"order": "by_witness_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes (assuming bob
, alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witness_votes",
"params": {
"start": ["bob", "alice"],
"limit": 10,
"order": "by_witness_account"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_witnesses
by_name
-start
requires value:account
by_vote_name
-start
requires 2 values:votes
,account
by_schedule_time
-start
requires 2 values:virtual_scheduled_time
,account
To list the first 10 witnesses ordered by name
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": "",
"limit": 10,
"order": "by_name"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of witnesses (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": "alice",
"limit": 10,
"order": "by_name"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 witnesses ordered by votes
, account
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": [0, ""],
"limit": 10,
"order": "by_vote_name"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of witnesses (assuming 0
, alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": [0, "alice"],
"limit": 10,
"order": "by_vote_name"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 witnesses ordered by virtual_scheduled_time
, account
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": [0, ""],
"limit": 10,
"order": "by_schedule_time"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of witnesses (assuming 473718186844702107410533306
, alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_witnesses",
"params": {
"start": ["473718186844702107410533306", "alice"],
"limit": 10,
"order": "by_schedule_time"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_proposal_votes
by_voter_proposal
-start
requires 2 values:voter
,proposal_id
by_proposal_voter
-start
requires 2 values:proposal_id
,voter
To list the first 10 votes ordered ascending by voter
, proposal_id
, filtered by status active
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposal_votes",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_voter_proposal",
"order_direction": "ascending",
"status": "active"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposal_votes",
"params": {
"start": ["alice", 99],
"limit": 10,
"order": "by_voter_proposal",
"order_direction": "ascending",
"status": "active"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 votes ordered ascending by proposal_id
, voter
filtered by status active
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposal_votes",
"params": {
"start": [0, ""],
"limit": 10,
"order": "by_proposal_voter",
"order_direction": "ascending",
"status": "active"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of votes (assuming 99
, alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposal_votes",
"params": {
"start": [99, "alice"],
"limit": 10,
"order": "by_proposal_voter",
"order_direction": "ascending",
"status": "active"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_proposals
by_creator
-start
requires 2 values:creator
,proposal_id
by_start_date
-start
requires 2 values:timestamp
,proposal_id
by_end_date
-start
requires 2 values:timestamp
,proposal_id
by_total_votes
-start
requires 2 values:vests
,proposal_id
To list the first 10 proposals ordered ascending by creator
, proposal_id
, filtered by status active
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": ["", 0],
"limit": 10,
"order": "by_creator",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of proposals (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": ["alice", 99],
"limit": 10,
"order": "by_creator",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 proposals ordered ascending by start_date
, proposal_id
, filtered by status active
(identical pattern to by_end_date
):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": ["1970-01-01T00:00:00", 0],
"limit": 10,
"order": "by_start_date",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of proposals (assuming 2019-09-12T00:00:00
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": ["2019-09-12T00:00:00", 99],
"limit": 10,
"order": "by_start_date",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 proposals ordered ascending by vests
, proposal_id
, filtered by status active
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": [0, 0],
"limit": 10,
"order": "by_total_votes",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of proposals (assuming 1272060680484393
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_proposals",
"params": {
"start": ["1272060680484393", 99],
"limit": 10,
"order": "by_total_votes",
"order_direction": "ascending",
"status":
"active"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_smt_contributions
by_symbol_id
-start
must be an empty array or consist ofsymbol
andid
by_symbol_contributor
-start
must be an empty array or consist ofcontributor
,symbol
andcontribution_id
To list the first 10 contributions ordered by symbol, id:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_contributions",
"params": {
"start": [],
"limit": 10,
"order": "by_symbol_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of contributions (assuming {"nai": "@@422838704", "decimals": 0}
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_contributions",
"params": {
"start": [{"nai": "@@422838704", "decimals": 0}, 0],
"limit": 10,
"order": "by_symbol_id"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 contributions ordered by symbol, id:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_contributions",
"params": {
"start": [],
"limit": 10,
"order": "by_symbol_contributor"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of contributions (assuming {"nai": "@@422838704", "decimals": 0}
, alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_contributions",
"params": {
"start": [{"nai": "@@422838704", "decimals": 0}, "alice", 99],
"limit": 10,
"order": "by_symbol_contributor"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_smt_token_emissions
by_symbol_time
-start
must be an empty array or consist ofsymbol
andtimestamp
To list the first 10 emissions ordered by symbol, timestamp:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_token_emissions",
"params": {
"start": [],
"limit": 10,
"order": "by_symbol_time"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of emissions (assuming {"nai": "@@422838704", "decimals": 0}
, "2019-08-07T16:54:03"
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_token_emissions",
"params": {
"start": [{"nai": "@@422838704", "decimals": 0}, "2019-08-07T16:54:03"],
"limit": 10,
"order": "by_symbol_time"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
database_api.list_smt_tokens
by_symbol
-start
must be asymbol
by_control_account
-start
must be anaccount
or an array containing anaccount
andsymbol
To list the first 10 tokens ordered by symbol:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_tokens",
"params": {
"start": {},
"limit": 10,
"order": "by_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of tokens (assuming {"nai": "@@422838704", "decimals": 0}
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_tokens",
"params": {
"start": {"nai": "@@422838704", "decimals": 0},
"limit": 10,
"order": "by_symbol"
},
"id": 1
}' https://api.hive.blog | jq
To list the first 10 tokens ordered by control account:
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_tokens",
"params": {
"start": "",
"limit": 10,
"order": "by_control_account"
},
"id": 1
}' https://api.hive.blog | jq
To list the next page of tokens (assuming alice
, {"nai": "@@422838704", "decimals": 0}
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "database_api.list_smt_tokens",
"params": {
"start": ["alice", {"nai": "@@422838704", "decimals": 0}],
"limit": 10,
"order": "by_control_account"
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
follow_api.get_account_reputations
To list the first 10 objects:
curl -s --data '{
"jsonrpc": "2.0",
"method": "follow_api.get_account_reputations",
"params":{
"account_lower_bound": "",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "follow_api.get_account_reputations",
"params":{
"account_lower_bound": "alice",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
reputation_api.get_account_reputations
To list the first 10 objects ordered by account
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "reputation_api.get_account_reputations",
"params": {
"account_lower_bound": "",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "reputation_api.get_account_reputations",
"params": {
"account_lower_bound": "alice",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
tags_api.get_discussions_by_author_before_date
Note: The before_date
param is completely ignored. This method is similar
to get_discussions_by_blog
but does not serve reblogs.
To list the first 10 objects for author
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "tags_api.get_discussions_by_author_before_date",
"params": {
"author": "alice",
"start_permlink": "",
"before_date": "",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice-permlink
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "tags_api.get_discussions_by_author_before_date",
"params": {
"author": "alice",
"start_permlink": "alice-permlink",
"before_date": "",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_account_history
See: account_history_api.get_account_history
Also see: API Definition
condenser_api.get_blog
To list the first 10 objects for account
, ordered by entry_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_blog",
"params": {
"account": "alice",
"start_entry_id": 0,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_blog",
"params": {
"account": "alice",
"start_entry_id": 99,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_blog_entries
To list the first 10 objects for account
, ordered by entry_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_blog_entries",
"params": {
"account": "alice",
"start_entry_id": 0,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_blog_entries",
"params": {
"account": "alice",
"start_entry_id": 99,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_discussions_by_author_before_date
See: tags_api.get_discussions_by_author_before_date
Also see: API Definition
condenser_api.get_feed
To list the first 10 objects for account
, ordered by entry_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenseer_api.get_feed",
"params": {
"account": "alice",
"start_entry_id": 0,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenseer_api.get_feed",
"params": {
"account": "alice",
"start_entry_id": 99,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_feed_entries
To list the first 10 objects for account
, ordered by entry_id
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_feed_entries",
"params": {
"account": "alice",
"start_entry_id": 0,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, 99
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_feed_entries",
"params": {
"account": "alice",
"start_entry_id": 99,
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_followers
To list the first 10 objects for account
, ordered by follower
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_followers",
"params": {
"account": "alice",
"start": "",
"type": "blog",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, bob
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_followers",
"params": {
"account": "alice",
"start": "bob",
"type": "blog",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_following
To list the first 10 objects for account
, ordered by followed
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_following",
"params": {
"account": "alice",
"start": "",
"type": "blog",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
To list the next page (assuming alice
, bob
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_following",
"params": {
"account": "alice",
"start": "bob",
"type": "blog",
"limit": 10
},
"id": 1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_replies_by_last_update
To list the first 10 objects for author
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_replies_by_last_update",
"params": {
"start_author": "alice",
"start_permlink": "",
"limit": 10
},
"id":1
}' https://api.hive.blog | jq
To list the next page (assuming alice-permlink
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_replies_by_last_update",
"params": {
"start_author": "alice",
"start_permlink": "alice-permlink",
"limit": 10
},
"id":1
}' https://api.hive.blog | jq
Also see: API Definition
condenser_api.get_vesting_delegations
See: database_api.list_vesting_delegations
Also see: API Definition
condenser_api.get_trending_tags
To list the first 10 objects for tags
:
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_trending_tags",
"params": {
"start_tag": null,
"limit": 10
},
"id":1
}' https://api.hive.blog | jq
To list the next page (assuming photography
is the last entry in the previous page):
curl -s --data '{
"jsonrpc": "2.0",
"method": "condenser_api.get_trending_tags",
"params": {
"start_tag": "photography",
"limit": 10
},
"id":1
}' https://api.hive.blog | jq
Also see: API Definition