Hive Developer Portal
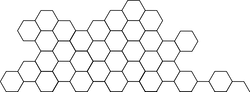
Using Keys Securely
Learn how the Beem python library handles transaction signing with Hive user’s key and how to securely manage your private keys.
Full, runnable src of Using Keys Securely can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-01_using_keys_securely.zip).
Intro
The Beem library has two ways to handle your keys. One is from source code, another one is through command line interface called beem
. beempy
cli is installed by default when you install beem library on your machine.
Note, the hive-python
library is out of date, but can be used in a pinch. For these tutorials, beem
is recommended.
Steps
- App setup - Library install and import
- Key usage example - Example showing how to import keys
1. App setup
In this tutorial we are only using beem
package - beem library.
# initialize Hive class
from beem import Hive
# defining private keys inside source code is not secure way but possible
h = Hive(keys=['<private_posting_key>', '<private_active_key>'])
a = Account('demo', blockchain_instance=h)
Last line from above snippet shows how to define private keys for account that’s going to transact using script.
2. Key usage example
After defining private keys inside Hive class, we can quickly sign any transaction and broadcast it to the network.
# above will allow accessing Commit methods such as
# demo account sending 0.001 HIVE to demo1 account
a.transfer('demo1', '0.001', 'HIVE', memo='memo text')
Above method works but it is not secure way of handling your keys because you have entered your keys within source code that you might leak accidentally. To avoid that, we can use CLI - command line interface beempy
.
You can type following to learn more about beempy
commands:
beempy -h
beempy
lets you leverage your BIP38 encrypted wallet to perform various actions on your accounts.
The first time you use beem, you will be prompted to enter a password. This password will be used to encrypt the beem wallet, which contains your private keys.
You can import your Hive username with following command:
beempy importaccount username
Next you can import individual private keys:
beempy addkey
That’s it, now that your keys are securely stored on your local machine, you can easily sign transaction from any of your Python scripts by using defined keys.
# if private keys are not defined
# accessing Wallet methods are also possible and secure way
h.wallet.getActiveKeyForAccount('demo')
Above line fetches private key for user demo
from local machine and signs transaction.
beempy
also allows you to sign and broadcast transactions from terminal. For example:
beempy transfer --account <account_name> <recipient_name> 1 HIVE memo
would sign and broadcast transfer operation,
beempy upvote --account <account_name> link
would sing and broadcast vote operation, etc.
That’s it!
Final code:
# initialize Hive class
from beem import Hive
from beem.account import Account
# defining private keys inside source code is not secure way but possible
h = Hive(keys=['<private_posting_key>', '<private_active_key>'])
a = Account('demo', blockchain_instance=h)
# above will allow accessing Commit methods such as
# demo account sending 0.001 HIVE to demo1 account
a.transfer('demo1', '0.001', 'HIVE', memo='memo text')
# if private keys are not defined
# accessing Wallet methods are also possible and secure way
h.wallet.getActiveKeyForAccount('demo')
To Run the tutorial
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/01_using_keys_securely
pip install -r requirements.txt
python index.py
- After a few moments, you should see output in terminal/command prompt screen.