Hive Developer Portal
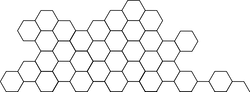
Get Posts
This example will output posts depending on which category is provided as the arguments.
Full, runnable src of Get Posts can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-04_get_posts.zip).
This tutorial will explain and show you how to access the Hive blockchain using the beem library to fetch list of posts filtered by a filter and tag.
Intro
In Hive there are built-in filters trending
, hot
, created
, active
, promoted
etc. which helps us to get list of posts. get_discussions_by_trending(query)
, get_discussions_by_hot(query)
, get_discussions_by_created(query)
, etc. functions are built-in into beem
that we are going to use throughout all Python tutorials.
Also see:
Steps
- App setup - Library install and import
- Filters list - List of filters to select from
- Query details - Form a query
- Print output - Print results in output
1. App setup
In this tutorial we use 3 packages, pick
- helps us to select filter interactively. beem
- hive library, interaction with Blockchain. pprint
- print results in better format.
First we import all three library and initialize Hive class
import pprint
from pick import pick
# initialize Hive class
from beem import Hive
h = Hive()
2. Filters list
Next we will make list of filters and setup pick
properly.
title = 'Please choose filter: '
#filters list
options = ['trending', 'hot', 'active', 'created', 'promoted']
# get index and selected filter name
option, index = pick(options, title)
This will show us list of filters to select in terminal/command prompt. And after selection we will get index and filter name to index
and option
variables.
3. Query details
Next we will form a query. In Hive,
- You can add a tag to filter the posts that you receive from the server
- You can also limit the amount of results you would like to receive from the query
q = Query(limit=2, tag="")
.
.
.
posts = {
0: d.get_discussions('trending', q, limit=2),
1: d.get_discussions('hot', q, limit=2),
2: d.get_discussions('active', q, limit=2),
3: d.get_discussions('created', q, limit=2),
4: d.get_discussions('promoted', q, limit=2)
}
Above code shows example of query and simple list of function that will fetch post list with user selected filter.
4. Print output
Next, we will print result, post list and selected filter name.
# print post list for selected filter
pprint.pprint(posts[index])
pprint.pprint("Selected: "+option)
The example of result returned from the service as objects:
1. <Comment @lukewearechange/unbelievable-how-are-they-getting-away-with-this>
2. <Comment @jennyzer/iniciativa-juegos-de-mi-infancia-or-or-games-of-my-childhood-initiative>
'Selected: hot'
From this result you have access to everything associated to the posts including additional metadata which is a JSON
string (that must be decoded to use), active_votes
info, post title, body, etc. details that can be used in further development of application with Python.
Final code:
import pprint
from pick import pick
# initialize Hive class
from beem import Hive
from beem.discussions import Query, Discussions
h = Hive()
title = 'Please choose filter: '
#filters list
options = ['trending', 'hot', 'active', 'created', 'promoted']
# get index and selected filter name
option, index = pick(options, title)
q = Query(limit=2, tag="")
d = Discussions()
count = 0
#post list for selected query
posts = {
0: d.get_discussions('trending', q, limit=2),
1: d.get_discussions('hot', q, limit=2),
2: d.get_discussions('active', q, limit=2),
3: d.get_discussions('created', q, limit=2),
4: d.get_discussions('promoted', q, limit=2)
}
# print post list for selected filter
for p in posts[index]:
print(("%d. " % (count + 1)) + str(p))
count += 1
pprint.pprint("Selected: " + option)
To Run the tutorial
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/04_get_posts
pip install -r requirements.txt
python index.py
- After a few moments, you should see output in terminal/command prompt screen.