Hive Developer Portal
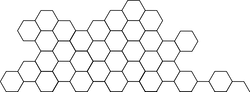
Get Post Details
This tutorial fetches the contents of a single post and explains all data related to that post.
Full, runnable src of Get Post Details can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-05_get_post_details.zip).
We will explain and show you how to access the Hive blockchain using the beem library to fetch list of posts filtered by a filter and tag.
Intro
Hive python library has built-in function to get details of post with author and permlink as an argument. Since we don’t have predefined post or author/permlink. We will fetch post list from previous tutorial and give option to choose one option/post to get its details. get_content
function fetches latest state of the post and delivers its details. Note that get_discussions_by_created
filter is used for fetching 5 posts which by default contains details of each post, but for purpose of this tutorial we will showcase get_content
function to fetch details.
Also see:
Steps
- App setup - Library install and import
- Post list - List of posts to select from created filter
- Post details - Get post details for selected post
- Print output - Print results in output
1. App setup
In this tutorial we use 3 packages, pick
- helps us to select filter interactively. beem
- hive library, interaction with Blockchain. pprint
- print results in better format.
First we import all three library and initialize Hive class
import pprint
from pick import pick
# initialize Hive class
from beem import Hive
from beem.discussions import Query, Discussions
from beem.comment import Comment
h = Hive()
2. Post list
Next we will fetch and make list of posts and setup pick
properly.
q = Query(limit=2, tag="")
d = Discussions()
#post list for selected query
posts = d.get_discussions('created', q, limit=2)
title = 'Please choose post: '
options = []
#posts list
for post in posts:
options.append(post["author"] + '/' + post["permlink"])
# get index and selected filter name
option, index = pick(options, title)
This will show us list of posts to select in terminal/command prompt. And after selection we will get index and post name to index
and option
variables.
3. Post details
Next we will fetch post details with get_content
. By default get_discussions_by_created
function already contains post details, but for this tutorial purpose we will ignore all other fields but only use author
and permlink
fields to fetch fresh post details.
details = Comment(option)
4. Print output
Next, we will print result, details of selected post.
# print post body for selected post
pprint.pprint('Depth: ' + str(details.depth))
pprint.pprint('Author: ' + details.author)
pprint.pprint('Category: ' + details.category)
pprint.pprint('Body: ' + details.body)
Also see: beem.comment.Comment
The example of result returned from the service:
'Depth: 0'
'Author: hiveio'
'Category: hive'
('Body: '
'\n'
'\n'
.
.
.
From this result you have access to everything associated to the post including additional metadata which is a JSON
string (e.g.; json()["created"]
), active_votes
(see: beem.comment.Comment.get_vote_with_curation) info, post title, body, etc. details that can be used in further development of applications with Python.
id
- Unique identifier that is mostly an implementation detail (best to ignore). To uniquely identify content, it's best to useauthor/permlink
.author
- The author account name of the content.permlink
- Permanent link of the content, must be unique in the scope of theauthor
.category
- The main category/tag this content belongs to.root_author
- Used in conbination withroot_permlink
.root_permlink
- In conbination withroot_author
, distinctly references discussion this content is part of.parent_author
- Parent author, in case this content is a comment (reply).parent_permlink
- Parent permanent link, this will be the same ascategory
for posts and will contain thepermlink
of the content being replied to in the case of a comment.title
- Title of the content.body
- Body of the content.json_metadata
- JSON metadata that holds extra information about the content. Note: The format for this field is not guaranteed to be valid JSON.last_update
- The date and time of the last update to this content.created
- The date and time this content was created.active
- The last time this content was "touched" by voting or reply.last_payout
- Time of last payout.depth
- Used to track max nested depth.children
- Used to track the total number of children, grandchildren, etc. ...net_rshares
- Reward is proportional to liniar rshares, this is the sum of all votes (positive and negative reward sum)abs_rshares
- This was used to track the total absolute weight of votes for the purpose of calculatingcashout_time
.vote_rshares
- Total positive rshares from all votes. Used to calculate delta weights. Needed to handle vote changing and removal.children_abs_rshares
- This was used to calculate cashout time of a discussion.cashout_time
- 7 days from thecreated
date.max_cashout_time
- Unused.total_vote_weight
- The total weight of voting rewards, used to calculate pro-rata share of curation payouts.reward_weight
- Weight/percent of reward.total_payout_value
- Tracks the total payout this content has received over time, measured in the debt asset.curator_payout_value
- Tracks the curator payout this content has received over time, measured in the debt asset.author_rewards
- Tracks the author payout this content has received over time, measured in the debt asset.net_votes
- Net positive votesroot_comment
- ID of the original content.max_accepted_payout
- Value of the maximum payout this content will receive.percent_hbd
- The percent of Hive Dollars to key, unkept amounts will be received as HIVE Power.allow_replies
- Allows content to disable replies.allow_votes
- Allows content to receive votes.allow_curation_rewards
- Allows curators of this content receive rewards.beneficiaries
- The list of up to 8 beneficiary accounts for this content as well as the percentage of the author reward they will receive in HIVE Power.url
- The end of the url to this content.root_title
- Title of the original content (useful in replies).pending_payout_value
- Pending payout amount if 7 days has not yet elapsed.total_pending_payout_value
- Total pending payout amount if 7 days has not yet elapsed.active_votes
- The entire voting list array, including upvotes, downvotes, and unvotes; used to calculatenet_votes
.replies
- Unused.author_reputation
- Author's reputation.promoted
- If post is promoted, how much has been spent on promotion.body_length
- Total content length.reblogged_by
- Unused.
Final code:
import pprint
from pick import pick
# initialize Hive class
from beem import Hive
from beem.discussions import Query, Discussions
from beem.comment import Comment
h = Hive()
q = Query(limit=2, tag="")
d = Discussions()
#post list for selected query
posts = d.get_discussions('created', q, limit=2)
title = 'Please choose post: '
options = []
#posts list
for post in posts:
options.append(post["author"] + '/' + post["permlink"])
# get index and selected filter name
option, index = pick(options, title)
details = Comment(option)
# print post body for selected post
# Also see: https://beem.readthedocs.io/en/latest/beem.comment.html#beem.comment.Comment
pprint.pprint('Depth: ' + str(details.depth))
pprint.pprint('Author: ' + details.author)
pprint.pprint('Category: ' + details.category)
pprint.pprint('Body: ' + details.body)
To Run the tutorial
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/05_get_post_details
pip install -r requirements.txt
python index.py
- After a few moments, you should see output in terminal/command prompt screen.