Hive Developer Portal
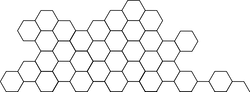
Search Accounts
How to call a list of user names from the Hive blockchain
Full, runnable src of Search Accounts can be downloaded as part of: tutorials/javascript (or download just this tutorial: devportal-master-tutorials-javascript-15_search_accounts.zip).
This tutorial will show the method of capturing a queried user name, matching that to the hive database and then displaying the matching names. This tutorial will be run on the production server
.
Intro
We are using the call
function provided by the dhive
library to pull user names from the hive blockchain. The information being pulled is dependent on two variables:
- The max number of user names that needs to be displayed by the search
- The string representing the first characters of the user name you are searching for
A simple HTML interface is used to both capture the string query as well as display the completed search. The layout can be found in the “index.html” file.
Also see:
Steps
- Configure connection Configuration of
dhive
to use the proper connection and network. - Collecting input variables Assigning and collecting the necessary variables
- Blockchain query Finding the data on the blockchain based on the inputs
- Output Displaying query results
1. Configure connection
Below we have dhive
pointing to the production network with the proper chainId, addressPrefix, and endpoint. There is a public/app.js
file which holds the Javascript segment of this tutorial. In the first few lines we define the configured library and packages:
const dhive = require('@hiveio/dhive');
let opts = {};
//connect to production server
opts.addressPrefix = 'STM';
opts.chainId =
'beeab0de00000000000000000000000000000000000000000000000000000000';
//connect to server which is connected to the network/production
const client = new dhive.Client('https://api.hive.blog');
2. Collecting input variables
Next we assign the max number of lines that will be returned by the query. We also use getElementById
to retrieve the requested user name for searching from the HTML interface. The max
value can very easily also be attained from the HTML side simply by adding another input line in index.html
and a second getElementById
line.
const max = 10;
window.submitAcc = async () => {
const accSearch = document.getElementById("username").value;
3. Blockchain query
The next step is to pull the user names from the blockchain that matches the “username” variable that was retrieved. This is done using the database.call
function and assigning the result to an array.
const _accounts = await client.database.call('lookup_accounts',[accSearch, max]);
console.log(`_accounts:`, _accounts);
4. Output
Finally we create a list from the “_accounts” array generated in step 3.
document.getElementById('accList').innerHTML = _accounts.join('<br>');
}
Final code:
const dhive = require('@hiveio/dhive');
let opts = {};
//connect to production server
opts.addressPrefix = 'STM';
opts.chainId =
'beeab0de00000000000000000000000000000000000000000000000000000000';
//connect to server which is connected to the network/production
const client = new dhive.Client('https://api.hive.blog');
//submitAcc function from html input
const max = 10;
window.submitAcc = async () => {
const accSearch = document.getElementById("username").value;
const _accounts = await client.database.call('lookup_accounts',[accSearch, max]);
console.log(`_accounts:`, _accounts);
//disply list of account names with line breaks
document.getElementById('accList').innerHTML = _accounts.join('<br>');
}
To run this tutorial
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/javascript/15_search_accounts
npm i
npm run dev-server
ornpm run start
- After a few moments, the server should be running at http://localhost:3000/